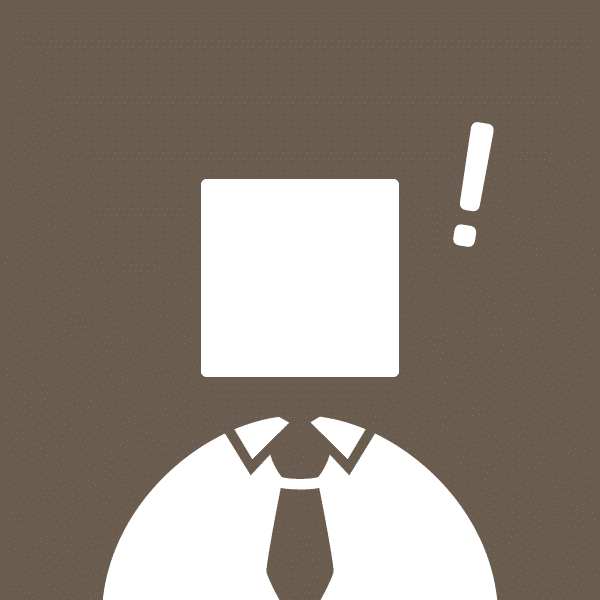
C#でJson使うなら絶対にライブラリインストールした方がいいよ!!
と激推しされたので。調べてみたらNewtonsoftの方がMicrosoftが用意しているライブラリよりも便利なだけではなく速いらしい。
本当に早くなるのなら追加機能とか関係なくJson使うなら絶対最初にインストールすべきだよね、というわけで今回検証してみます。
Newtonsoft.Jsonライブラリとは
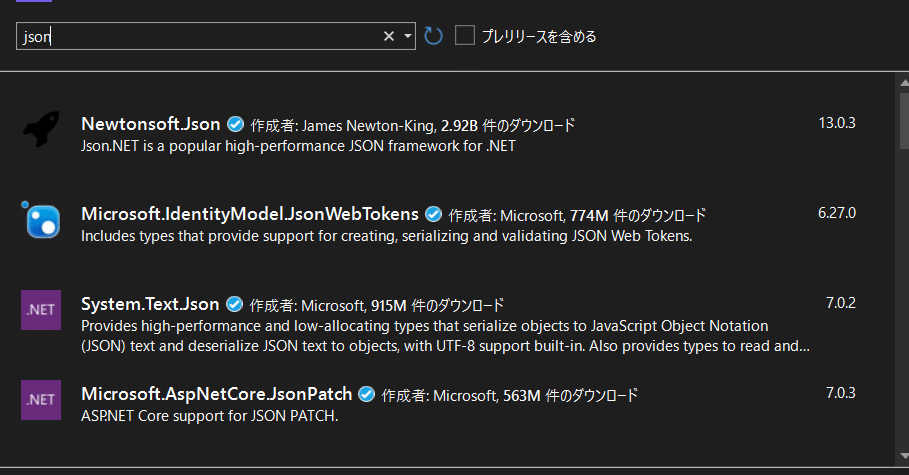
読んで見てそのまま、Jsonを扱うためのライブラリです。
サンプルコード
今回作成したコードはこんな感じ。
Jsonファイルを読み込んで、デシリアライズしてシリアライズしてコンソール出力。
テスト用のデータはJSON GENERATORで4000行くらいのデータを作りました。便利。
using Newtonsoft.Json;
using System;
using System.IO;
using System.Text;
using System.Collections.Generic;
namespace JsonSample
{
class Program
{
static void Main()
{
List<Person> person = new List<Person>();
using (var reader = new StreamReader(@"C:\jikken\jikken.json",
Encoding.GetEncoding("utf-8")))
{
person = JsonConvert.DeserializeObject<List<Person>>(reader.ReadToEnd());
}
string output = JsonConvert.SerializeObject(person);
Console.WriteLine(output);
}
public class Person
{
public string _id { get; set; }
public int index { get; set; }
public string guid { get; set; }
public bool isActive { get; set; }
public string balance { get; set; }
public string picture { get; set; }
public int age { get; set; }
public string eyeColor { get; set; }
public string name { get; set; }
public string gender { get; set; }
public string company { get; set; }
public string email { get; set; }
public string phone { get; set; }
public string address { get; set; }
public string about { get; set; }
public string registered { get; set; }
public string latitude { get; set; }
public string longitude { get; set; }
public string[] tags { get; set; }
public List<friends> friends { get; set; }
public string greeting { get; set; }
public string favoriteFruit { get; set; }
}
public class friends
{
public int id { get; set; }
public string name { get; set; }
}
}
}
Jsonをデシリアライズする
どの箇所でデシリアライズか説明不要だとは思いますが念のため。18行目です。
person = JsonConvert.DeserializeObject<List<Person>>(reader.ReadToEnd());
そしてこの式の実行時間を計測するとこんな感じ。

4000行のテストデータを読み込むのにかかった時間は0.1715秒でした。
Jsonをシリアライズする
シリアライズは21行目でしています。
string output = JsonConvert.SerializeObject(person);

0.0267秒でした。
速度を比較してみる
今回はSystem.Text.Jsonと速度を比較してみようと思います。こちらも使用時にNugetでパッケージのインストールが必要なやつです。
System.Text.Json用に作成したコードはこんな感じ。
using System.Text.Json;
using System.Text.Json.Serialization;
using System;
using System.IO;
using System.Text;
using System.Collections.Generic;
namespace JsonSample
{
class Program
{
static void Main()
{
List<Person> person = new List<Person>();
using (var reader = new StreamReader(@"C:\jikken\jikken.json",
Encoding.GetEncoding("utf-8")))
{
person = JsonSerializer.Deserialize<List<Person>>(reader.ReadToEnd());
}
string output = JsonSerializer.Serialize(person);
Console.WriteLine(output);
}
public class Person
{
public string _id { get; set; }
public int index { get; set; }
public string guid { get; set; }
public bool isActive { get; set; }
public string balance { get; set; }
public string picture { get; set; }
public int age { get; set; }
public string eyeColor { get; set; }
public string name { get; set; }
public string gender { get; set; }
public string company { get; set; }
public string email { get; set; }
public string phone { get; set; }
public string address { get; set; }
public string about { get; set; }
public string registered { get; set; }
public string latitude { get; set; }
public string longitude { get; set; }
public string[] tags { get; set; }
public List<friends> friends { get; set; }
public string greeting { get; set; }
public string favoriteFruit { get; set; }
}
public class friends
{
public int id { get; set; }
public string name { get; set; }
}
}
}
本当にシリアライズとデシリアライズの部分を変えただけです。
そして、気になる結果がこちら。
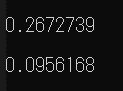
デシリアライズにかかったのは0.2672秒、シリアライズにかかったのは0.0956秒でした。
シリアライズの方は実行時間に結構ばらつきがあったので何回か計測しました。それでも0.04秒を切ることはなかったのでNewtonsoft.Jsonの方が処理速度が速いことが分かります。
結果
Newtonsoft.Jsonの方が速いことが分かりました。
デシリアライズ | シリアライズ | |
System.Text.Json | 0.2672 | 0.0956 |
Newtonsoft.Json | 0.1715 | 0.0267 |
テスト用に同じJSONファイルを読み込ませたのにSystem.Text.Jsonだけエラーを吐いたりなかなか謎でした。エラーになった原因については以下記事にまとめています。